When working in IDA to reverse games, you can end up with lots of dummy/empty labels, that are auto generated when doing offset work. Here’s my script to remove them.
First how it happens.
You find a value you are interested in setting to a offset
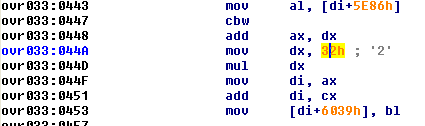
And then you right click and go down the offset menu, and review the choices.
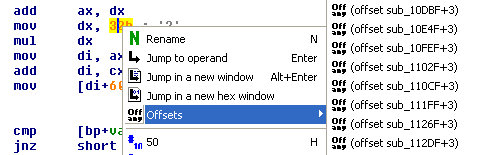
This just created a dummy label on every segment at offset 32h so it could display it to you.
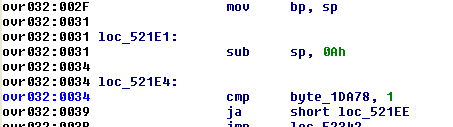
Now you can remove these manually by selecting the line and pressing n then empting the name, and pressing ok.
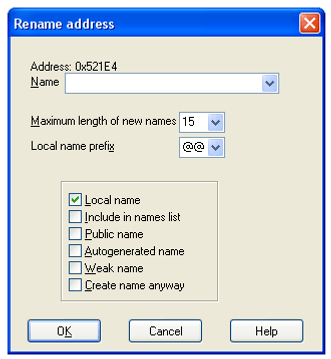
But that’s painful if you have hundreds of dummy labels. Roll on the power of IDC files, and lets get rid of those.
#include <idc.idc>
static main()
{
auto seg, loc, flags;
auto count;
count = 0;
seg = FirstSeg();
while(seg != BADADDR )
{
loc = SegStart(seg);
while( loc < SegEnd(seg) )
{
flags = GetFlags(loc);
// Has a dummy label and no references, and not start of function, remove name
if( ((flags & ( FF_LABL | FF_REF)) == FF_LABL) & ((flags & FF_FUNC) == 0))
{
MakeNameEx(loc, "", 0);
count ++;
}
loc = loc + ItemSize(loc);
}
seg = NextSeg(seg);
}
Message("Removed %d empty labels\\n", count);
}
Now you can safely remove the non referenced auto labels. It leaves functions names, or those that don’t follow the auto label format loc_xxxxxx